Using the Google Maps library, you can create your own map-viewing Activity.
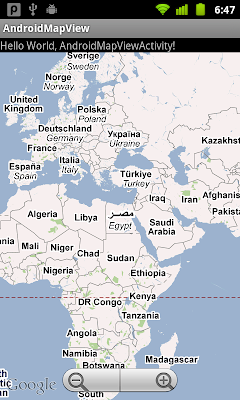
By default the Android SDK includes the Google APIs add-on, which in turn includes the Maps external library. The Google APIs add-on requires Android 1.5 SDK or later release.
Create a Android project with build target called "Google APIs".
Modify AndroidManifest.xml to include uses-library "com.google.android.maps", and grant permission of "android.permission.INTERNET".
To embed a MapView in your app, you have to obtain a Maps API key, refer to this post.
Modify main.xml to add com.google.android.maps.MapView, with your own Maps API Key.
Main activity, extends MapActivity.
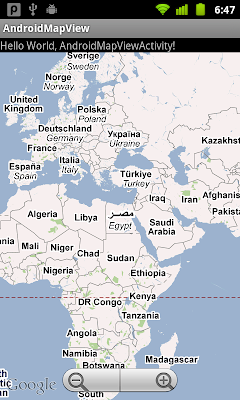
By default the Android SDK includes the Google APIs add-on, which in turn includes the Maps external library. The Google APIs add-on requires Android 1.5 SDK or later release.
Create a Android project with build target called "Google APIs".
Modify AndroidManifest.xml to include uses-library "com.google.android.maps", and grant permission of "android.permission.INTERNET".
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.AndroidMapView" android:versionCode="1" android:versionName="1.0"> <uses-sdk android:minSdkVersion="4" /> <application android:icon="@drawable/icon" android:label="@string/app_name"> <uses-library android:name="com.google.android.maps" /> <activity android:name=".AndroidMapViewActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> <uses-permission android:name="android.permission.INTERNET"/> </manifest>
To embed a MapView in your app, you have to obtain a Maps API key, refer to this post.
Modify main.xml to add com.google.android.maps.MapView, with your own Maps API Key.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <com.google.android.maps.MapView android:id="@+id/mapview" android:layout_width="fill_parent" android:layout_height="fill_parent" android:clickable="true" android:apiKey="Your Maps API key here" /> </LinearLayout>
Main activity, extends MapActivity.
package com.AndroidMapView; import com.google.android.maps.MapActivity; import com.google.android.maps.MapView; import android.os.Bundle; public class AndroidMapViewActivity extends MapActivity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); MapView mapView = (MapView) findViewById(R.id.mapview); mapView.setBuiltInZoomControls(true); } @Override protected boolean isRouteDisplayed() { // TODO Auto-generated method stub return false; } }
No comments:
Post a Comment