Layouts:
@ home.xml Layout: This layout is the welcome layout for Quick Calculator application. In this layout, a wallpaper is set as background of the layout. While we start this application, this layout runs for 3 seconds.
Listing 1: home.xml layout
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/calc" android:orientation="vertical" > <TextView android:layout_width="wrap_content" android:layout_height="35dp" android:layout_marginLeft="20dp" android:layout_marginTop="25dp" android:text="@string/app_name" android:textColor="#000" android:textSize="25sp" /> <TextView android:layout_width="wrap_content" android:layout_height="25dp" android:layout_marginLeft="70dp" android:layout_weight="0.04" android:text="@string/app_name_slogan" android:textColor="#000" android:textSize="15sp" /> </LinearLayout>
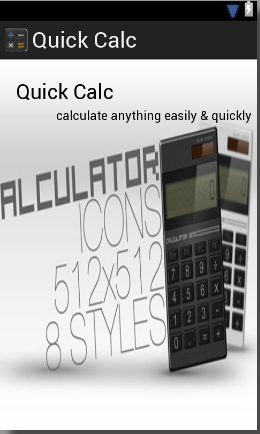
Figure 1: Quick Calculator
Java Class Files:
Every Android project contains a "res" (reources) folder. This folder is meant to comprise of number of subfolders.
Listing 2: calc.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".Home" android:background="#fff" > <EditText android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/disp" android:id = "@+id/display" android:hint="@string/dispHint" /> <LinearLayout android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:gravity="center" android:paddingTop="20dp"> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/seven" android:text="@string/seven" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/eight" android:text="@string/eight" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/nine" android:text="@string/nine" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/div" android:text="@string/div" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:gravity="center" android:paddingTop="20dp"> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/four" android:text="@string/four" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/five" android:text="@string/five" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/six" android:text="@string/six" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/mul" android:text="@string/mul" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:gravity="center" android:paddingTop="20dp"> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/one" android:text="@string/one" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/two" android:text="@string/two" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/three" android:text="@string/three" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/sub" android:text="@string/sub" /> </LinearLayout> <LinearLayout android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:gravity="center" android:paddingTop="20dp"> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/cancel" android:text="@string/cancel" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/zero" android:text="@string/zero" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/equal" android:text="@string/equal" /> <Button android:layout_width="55dp" android:layout_height="wrap_content" android:id = "@+id/add" android:text="@string/add" /> </LinearLayout> </LinearLayout>
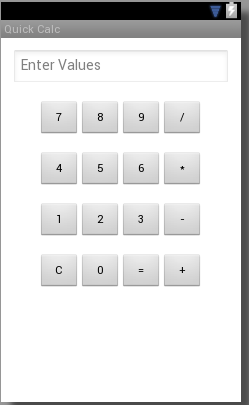
Figure 2: Quick Calculator
In this layout one EditText is used as display and rest of object are button objects, which are labeled from 1 to 0 natural numbers, mathematical operations, equal button, and reset button.
Two java classes are created in order to manage these two layouts for this application, the first class named home class run home.xml layout for 3 seconds using Thread concept and loads the next layout after 3 seconds by closing the running layout (home.xml).
Note: Layout is closed by calling method finish() and other layout is loaded using new Intent mechanism.
Listing 3: Home class defined in the Home.java file.
import android.app.Activity; import android.content.Intent; import android.os.Bundle; public class Home extends Activity{ @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); setContentView(R.layout.home); Thread th = new Thread(){ public void run(){ try{ sleep(1000); } catch(Exception e){ e.printStackTrace(); } finally{ onPause(); startActivity(new Intent("com.one.slate.CALC")); } } }; th.start(); } @Override public void onPause(){ super.onPause(); finish(); } }
This class extends an Activity class. Two methods of Activity class are overridden in this class, which are onCreate(Bundle) and onPause(). onCreate() method defines, which layout is to be loaded using method: setContentView(R.layout.home);
Next, it defines a new thread, which runs the layout for 3 seconds and load next activity layout by starting a new activity: startActivity(new Intent(“com.one.slate.CALC”));
Before starting a new activity, we should close running activity, if it will not be used in future. This can be achieved using onPause() method.
Listing 3: Calculator class defind into the Calc.java file.
import android.os.Bundle; import android.app.Activity; import android.text.Editable; import android.view.Menu; import android.view.View; import android.widget.Button; import android.widget.EditText; public class Calc extends Activity implements View.OnClickListener{ Button one, two, three, four, five, six, seven, eight, nine, zero, add, sub, mul, div, cancel, equal; EditText disp; int op1; int op2; String optr; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_home); one = (Button) findViewById(R.id.one); two = (Button) findViewById(R.id.two); three = (Button) findViewById(R.id.three); four = (Button) findViewById(R.id.four); five = (Button) findViewById(R.id.five); six = (Button) findViewById(R.id.six); seven = (Button) findViewById(R.id.seven); eight = (Button) findViewById(R.id.eight); nine = (Button) findViewById(R.id.nine); zero = (Button) findViewById(R.id.zero); add = (Button) findViewById(R.id.add); sub = (Button) findViewById(R.id.sub); mul = (Button) findViewById(R.id.mul); div = (Button) findViewById(R.id.div); cancel = (Button) findViewById(R.id.cancel); equal = (Button) findViewById(R.id.equal); disp = (EditText) findViewById(R.id.display); try{ one.setOnClickListener(this); two.setOnClickListener(this); three.setOnClickListener(this); four.setOnClickListener(this); five.setOnClickListener(this); six.setOnClickListener(this); seven.setOnClickListener(this); eight.setOnClickListener(this); nine.setOnClickListener(this); zero.setOnClickListener(this); cancel.setOnClickListener(this); add.setOnClickListener(this); sub.setOnClickListener(this); mul.setOnClickListener(this); div.setOnClickListener(this); equal.setOnClickListener(this); } catch(Exception e){ } } public void operation(){ if(optr.equals("+")){ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 + op2; disp.setText("Result : " + Integer.toString(op1)); } else if(optr.equals("-")){ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 - op2; disp.setText("Result : " + Integer.toString(op1)); } else if(optr.equals("*")){ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 * op2; disp.setText("Result : " + Integer.toString(op1)); } else if(optr.equals("/")){ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 / op2; disp.setText("Result : " + Integer.toString(op1)); } } @Override public void onClick(View arg0) { Editable str = disp.getText(); switch(arg0.getId()){ case R.id.one: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(two.getText()); disp.setText(str); break; case R.id.two: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(two.getText()); disp.setText(str); break; case R.id.three: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(three.getText()); disp.setText(str); break; case R.id.four: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(four.getText()); disp.setText(str); break; case R.id.five: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(five.getText()); disp.setText(str); break; case R.id.six: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(six.getText()); disp.setText(str); break; case R.id.seven: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(eight.getText()); disp.setText(str); break; case R.id.eight: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(nine.getText()); disp.setText(str); break; case R.id.nine: if(op2 != 0){ op2 = 0; disp.setText(""); } str = str.append(zero.getText()); disp.setText(str); break; case R.id.cancel: op1 = 0; op2 = 0; disp.setText(""); disp.setHint("Perform Operation :)"); break; case R.id.add: optr = "+"; if(op1 == 0){ op1 = Integer.parseInt(disp.getText().toString()); disp.setText(""); } else if(op2 != 0){ op2 = 0; disp.setText(""); } else{ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 + op2; disp.setText("Result : " + Integer.toString(op1)); } break; case R.id.sub: optr = "-"; if(op1 == 0){ op1 = Integer.parseInt(disp.getText().toString()); disp.setText(""); } else if(op2 != 0){ op2 = 0; disp.setText(""); } else{ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 - op2; disp.setText("Result : " + Integer.toString(op1)); } break; case R.id.mul: optr = "*"; if(op1 == 0){ op1 = Integer.parseInt(disp.getText().toString()); disp.setText(""); } else if(op2 != 0){ op2 = 0; disp.setText(""); } else{ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 * op2; disp.setText("Result : " + Integer.toString(op1)); } break; case R.id.div: optr = "/"; if(op1 == 0){ op1 = Integer.parseInt(disp.getText().toString()); disp.setText(""); } else if(op2 != 0){ op2 = 0; disp.setText(""); } else{ op2 = Integer.parseInt(disp.getText().toString()); disp.setText(""); op1 = op1 / op2; disp.setText("Result : " + Integer.toString(op1)); } break; case R.id.equal: if(!optr.equals(null)){ if(op2 != 0){ if(optr.equals("+")){ disp.setText(""); /*op1 = op1 + op2;*/ disp.setText("Result : " + Integer.toString(op1)); } else if(optr.equals("-")){ disp.setText("");/* op1 = op1 - op2;*/ disp.setText("Result : " + Integer.toString(op1)); } else if(optr.equals("*")){ disp.setText("");/* op1 = op1 * op2;*/ disp.setText("Result : " + Integer.toString(op1)); } else if(optr.equals("/")){ disp.setText("");/* op1 = op1 / op2;*/ disp.setText("Result : " + Integer.toString(op1)); } } else{ operation(); } } break; } } }
This class describe all the complete functionality for a calculator. This class extends Activity class and implements method of View.OnClickListener interface.
- onCreate(Bundle) method loads the calc.xml layout.
- operation() method define all the basic operation for calculator.
- onClick() method is implemented of OnClickListener interface, which handle all the clicking buttons of calculator application.
- These all buttons clicks are managed by switch case conditional statement, where case represents the layout attributes id and describe their functionality.
- setOnClickListener(this) points out click event defined as a switch case in the onClick() method respectively.
Listing 4: XML : AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.one.slate" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="17" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.one.slate.Home" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name="com.one.slate.Calc" android:label="@string/app_name" > <intent-filter> <action android:name="com.one.slate.CALC" /> <category android:name="android.intent.category.DEFAULT" /> </intent-filter> </activity> </application> </manifest>
The AndroidManifest.xml file is the main configuration file for Quick Calculator android application. This loads home layout by default using class Home.class, whose parameters are set into the activity name “.Home”. The next activity defines, how to load next layout (calc). This layout is loaded by Calc.class defined as parameters in this second activity.
These two activities are enclosed into the main xml tag , which describes information about the application representing, when an application starts.
Read more: http://mrbool.com/how-to-create-a-calculator-app-for-android/28100#ixzz31OB9bECx